If you find this helpful, please click the Google |


The <img/> Tag in HTML 5
The <img/>
tag puts an image on a web page by reading the binary image data from a separate file or external resource and including it inline with other content on the page. It is one of the embedded content tags in HTML. As embedded content, the image is read from the resource specified by the src attribute and dynamically inserted into the web page when it is rendered. For inline video content, see the <iframe> tag, which works almost like an img element except the content in the external resource is HTML code rather than binary image data.
In the <img/>
tag demo below, the image button on the left uses legacy image rollover techniques. The image button on the right uses more sophisticated animated rollover, but the animation may not be supported in all browsers yet.
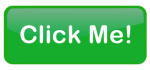
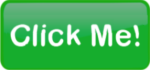
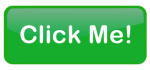
These are actual working examples of the <img> tag example code below, since this entire page is Valid HTML 5.
<img/> Tag Syntax
Rules for coding HTML img
elements
<body> ... ... phrasing content expected ...<img src="image-URL" alt="image description"/>... ... </body>
Rules for coding the HTML img element
Make sure you understand the difference between a tag and element and are familiar with the definitions of namespace and other HTML terms.
- Code the img element where phrasing content is expected.
- The img element consists of a standalone <img/> tag. The element name uses lower case letters and should be in the HTML namespace, which it will pick up automatically from the
xmlns
attribute on the <html> tag. - Code a
src
attribute with the URL of the image resource. - Code an
alt
attribute with a description of the image for use by non-visual clients such as auditory browsers and as an alternative in case the image resource is unavailable for some reason. - Include any other attributes of the <img> tag as appropriate.
- Since the img element is a void element, it should always be coded as a self-closing tag terminated with the delimiter string
/>
.
<img/> Content Model
Contents of the img element
Content: Empty. All properties are coded using attributes.
Since the <img/>
tag is a void element, it is not allowed to have any content, even HTML comments and therefore should always be coded as a self-closing standalone tag, ending with the delimiters />
rather than just >
(<img .../>
).
<img/> Tag Attributes
Attributes of the <img/> tag
global attributes | In addition to the local attributes of the <img/> tag below, any of the common HTML attributes can also be coded. |
width ,height |
|
src |
a URI reference that resolves to the URL of a two-dimensional image resource Use percent escape codes as explained in the URL-encoding tutorial for any special characters in the URI reference. If the value of the
If the protocol scheme, username, host name/IP address and port number are omitted the default is the current host - the same server as the base of the current document. If the path starts with a slash |
alt |
Provides alternative content when images are blocked or disabled or for users who are unable to view the image. See Difference between image alt text and image title below. |
ismap="ismap" |
Sets the value of the <img ismap> boolean attribute to true . Omitting it sets to false . |
usemap |
a URI reference that resolves to the URL of an image map Use percent escape codes as explained in the URL-encoding tutorial for any special characters in the URI reference. |
Difference between image alt text and image title
The image alt text in the <img alt> attribute provides alternative content when images are blocked or disabled or for users who are unable to view the image.
The image title in the global title attribute provides a description of the image and may be displayed in a pop-up tool tip when the mouse is held over the image.
<img/> Tag Examples
Examples of the <img/> tag in HTML 5
Simple <img/>
code
<img src="/images/html-5-logo.png" alt="HTML-5.com"/>
The src attribute and alt attribute are both required.
Image link
<a href="http://www.ExampleOnly.com/todos/take-out-trash.html" title="Take out the trash"> <img src="/images/trash-can.png" alt="trash can"/> </a>
When the a element and img element represent the same resource or event, the title attribute should be put on the outermost element, which in this case is the hypertext link created by the <a> tag. Since there is no title attribute on the <img> tag, the value of the title property of the img element will be inherited from the title property of the a element.
Link with image and text
<a href="http://www.ExampleOnly.com/todos/take-out-trash.html" title="Take out the trash"> <img src="/images/task-not-done.png" alt="task not done" title=""/> Take out the trash</a>
In this case, the a element represents a different resource (a task in a "To Do" list) than the image element (the status of the task). Therefore the title property of the img element should not be inherited from the <a> tag, which requires coding the title attribute on the <img/> tag. The value of the title property is an empty string since the <img alt> attribute provides the description of the image.
Clear after a floating image so headings do not flow around it
You can float an image with style="float: left" or style="float: right" then follow it with headings. If you try to position the headings below the image with style="clear: both", some browsers (Chrome, Safari) will move the headings below the image while other browsers (Firefox, Opera) will flow the headings around the image. For example:
<img src="/images/mathml.png" alt="" style="float: left; border: 1px solid gray; padding: 6px"/> <p>This is the text that flows to the right of the floating image.</p> <hgroup style="clear: both"> <h3>This Is A Heading That Should Appear After The Image</h3> <h4>It Is Followed By Another Heading That Is Just A Little Longer</h4> </hgroup>
View this in Firefox or Opera to see what happens:
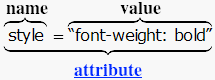
This is the text that flows to the right of the floating image.
This Is A Heading That Should Appear After The Image
It Is Followed By Another Heading That Is Just A Little Longer
To make it look consistent in all browsers, code display: block in the style
attribute to make sure the element with the clear: both style is rendered as a block element. Now that the position of the headings is correct, code a text-align: center style to center the headings if desired.
<img src="/images/mathml.png" alt="" style="float: left; border: 1px solid gray; padding: 6px"/> <p>This is the text that flows to the right of the floating image.</p> <hgroup style="display: block; clear: both; text-align: center"> <h3>This Is A Heading That Should Appear After The Image</h3> <h4>It Is Followed By Another Heading That Is Just A Little Longer</h4> </hgroup>
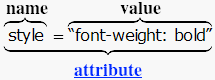
This is the text that flows to the right of the floating image.
This Is A Heading That Should Appear After The Image
It Is Followed By Another Heading That Is Just A Little Longer
Of course the styles can be put into a CSS Style Sheet, possibly with a class
selector.
Simple image rollover
(see "Click Me" image rollover demo on left above)
<img src="/images/click-me-normal.png" alt="img rollover demo" onmouseover="this.src='/images/click-me-hover.png'" onmouseout="this.src='/images/click-me-normal.png'" onclick="location.href='#examples'" />
Animated image rollover
(see "Click Me" image rollover demo on right above)
<div id="click-me-image-button"> <style scoped="scoped"> div#click-me-image-button img { -webkit-transition: opacity 250ms ease-in-out; -moz-transition: opacity 250ms ease-in-out; -o-transition: opacity 250ms ease-in-out; } img#ex1img1, img#ex1img2:hover { opacity: 1.0; } img#ex1img2, img#ex1img1:hover { opacity: 0; } </style> <img id="ex1img2" style="position: absolute" src="/images/click-me-hover.png" alt="" onclick="location.href='#examples'" /> <img id="ex1img1" src="/images/click-me-normal.png" alt=""/> </div>
Example of resizing image with link
<p style="text-align: center">Click this image to view the <video> tag demo<br/><br/> <a href="../video-tag/index.html#examples"> <img src="/media/deep-impact-movie.png" width="160" height="120" alt="picture from deep impact movie" /> </a> </p>
This example puts an image in an <a> tag that links to the HTML 5 <video> demo. Also shows how to resize an image; in this case the size of the image is changed from 320x240 to 160x120.
Image Fade-In Transition
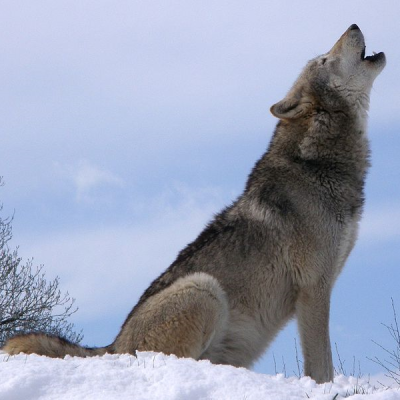
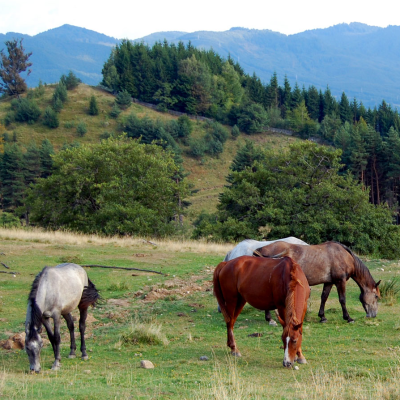
Example of rotated image with drop shadow
on HTML canvas with fallback to standard HTML image code
<canvas id="ex3canvas" width="215" height="170"> <img width="160" height="120" src="/media/deep-impact-movie.png" onload="ctx = document.getElementById('ex3canvas').getContext('2d'); ctx.rotate(-0.1); ctx.shadowOffsetX = 15; ctx.shadowOffsetY = 15; ctx.shadowBlur = 15; ctx.shadowColor = '#333333'; ctx.drawImage(this, 10, 35, 160, 120);" /> </canvas>
The preferred size for the image is coded in both the <img> tag attributes and the drawImage
function and the size of the canvas is slightly larger to allow for the extra space used for rotation and the drop shadow. In HTML 5 browsers, the width
and height
attributes on the <img> tag will be ignored. Browsers that do not support the HTML 5 <canvas> tag, should fall back to the content of the <canvas> tag, which is simply a standard <img> tag. If the width
and height
attributes were omitted, the image would only be resized in browsers drawing the image on the canvas with the drawImage
function.
Here is an actual working example of the code above. If the edges are jagged or the drop shadow is missing then compare how it looks in other browsers. (Do View Source to verify that this page is using the HTML 5 DOCTYPE. You can also verify it is Valid HTML 5 using the HTML Validator. Try using it to validate URLs with HTML examples from other places that claim to be HTML 5 web sites!)
Changes in HTML 5 - <img/> Tag
What's new in HTML 5
Differences between HTML 5 and earlier versions of HTML
The following attributes should not be coded on the <img> tag because they either have been deprecated or were never officially supported:
align
border
controls
dynsrc
hspace
loop
start
vspace
In ployglot HTML documents the <img/>
tag is coded as a self-closing tag the same as in the 2000-2010 Recommendations from the W3C HTML Working Group. Those specifications changed the ending delimiter to />
from >
in older recommendations. They also defined the HTML namespace for the img element type name and the names of other HTML element types.